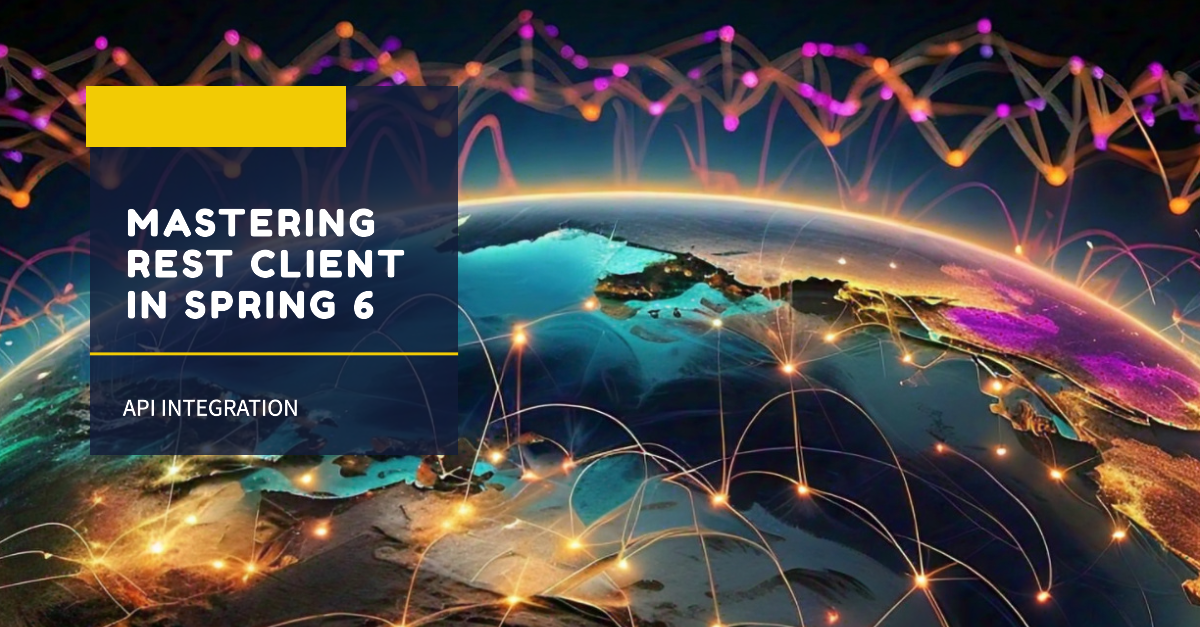
Introduction
Introduced in Spring 6, RestClient is a synchronous HTTP client designed with a modern and fluent API. It provides a streamlined abstraction over various HTTP libraries, making it easy to convert Java objects into HTTP requests and transform HTTP responses back into Java objects. In this blog post, we will explore the features of RestClient and demonstrate its usage by making API calls to OpenWeather to get weather information for a location.
Creating a RestClient
RestClient
can be created using its static create methods or a builder for more customization.
Default RestClient
RestClient defaultClient = RestClient.create();
Using Builder
Using builder allows to provide default values during RestClient intialisation. In our usecase we will set the base url and a default header for weather API
RestClient weatherClient = RestClient.builder() .baseUrl("https://api.openweathermap.org") .defaultHeader("request-id", "req1") .build();
Using the RestClient
Depending on the HTTP method to be used, methods get()
, post()
etc. in RestClient
can be called. In our case for the weather API, it is a GET call to the geo end point to get the location coordinates first.
So we will call RestClient.get
and then pass the URL to the uri
method.
String location = "Sydney"; String apiKey = "test-key"; // Register in openweathermap to get key String geoUrl = String.format("/geo/1.0/direct?q=%s&limit=1&appid=%s", location, apiKey); restClient.get().uri(geoUrl);
Retrieving data to Java object
After setting up the request, we can make the call and get the response by calling retrieve()
. The response body can be accessed using body(Class)
for standard types or body(ParameterizedTypeReference)
for parameterized types such as lists. The body
method transforms the response content into different types like String, classes, records etc.
String result = restClient.get() .uri("https://example") .retrieve() .body(String.class);
In our case, we will call a geolocation API to get the latitude and longitude of the location. The response will be a list of GeoData
objects.
public record GeoData(double lat, double lon) {} String location = "Sydney"; String apiKey = "test-key"; // Register in openweather to get key String geoUrl = String.format("/geo/1.0/direct?q=%s&limit=1&appid=%s", location, apiKey); List<GeoData> geoData = restClient.get() .uri(geoUrl) .retrieve() .body(new ParameterizedTypeReference<List<GeoData>>() {});
Now let us call the second API with latitude and longitude from this response
record WeatherData(Main main, List<Weather> weather); String weatherUrl = String.format("/geo/1.0/direct?data/2.5/weather? lat=%s&lon=%s&appid=%s",lat,lon, apiKey); WeatherData weather = restClient.get() .uri(weatherUrl) .retrieve() .body(WeatherData.class);
Similar to Get
calls, other HTTP methods like Post
can be invoked using the same way. Post
example given below
ResponseEntity<Void> response = restClient.post() .uri("https://crm.test.com/customer/new") .contentType(APPLICATION_JSON) .body(customer) .retrieve() .toBodilessEntity();
Error Handling
RestClient throws a subclass of RestClientException
when it receives a response with a 4xx or 5xx status code. This behavior can be modified using the onStatus
method.
WeatherData weather = restClient.get() .uri(weatherUrl) .retrieve() .onStatus(HttpStatusCode::is4xxClientError, (request, response) -> { throw new WeatherNotAvailableException(response.getStatusCode(), response.getHeaders()); }) .body(WeatherData.class);
Conclusion
In this article we learned how to call APIs using new fluent RestClient API.
To stay updated with the latest updates in Java and Spring follow us on youtube, linked in and medium.
Video Tutorial
To watch a detailed tutorial on RestClient, check out our YouTube video below:
Related Posts
Building a Smart Investment Portfolio Advisor with Java, Spring Boot, and LangChain4j
Learn how to build an AI-powered stock portfolio advisor using Java, Spring Boot, LangChain4j, and OpenAI/Ollama. This guide walks you through integrating AI into your application to provide real-time investment advice based on the latest stock data.
Building WebSocket Applications with Spring Boot
Learn how to build real-time applications using WebSockets in Spring Boot. This guide covers both simple WebSocket implementation and STOMP-based messaging, with practical examples of building a chat application.